Announcing CDK for Terraform 0.1
CDK for Terraform now supports Java and C# and has new collaboration features on Terraform Cloud.
In July 2020, we announced CDK for Terraform, a project that allows users to define infrastructure using programming languages such as Python and TypeScript while leveraging the hundreds of providers and thousands of module definitions provided by HashiCorp Terraform and the Terraform ecosystem.
Today, we are pleased to announce the release of CDK for Terraform 0.1. This release contains various enhancements and the addition of C# and Java programming language support from the previous releases. The additions also include features that make collaborating on CDK for Terraform projects easier using Terraform Cloud. This release brings us closer to a beta version of CDK for Terraform.
» Support for C#
CDK for Terraform 0.1 includes C# support that allows users to write CDK constructs to define infrastructure in C# and generate Terraform JSON configuration to provision that infrastructure.
using System;
using Constructs;
using Hashicorp.Cdktf;
using aws;
namespace MyCompany.MyApp
{
class MyApp : TerraformStack
{
public MyApp(Construct scope, string id) : base(scope, id)
{
new AwsProvider(this, "AWS", new AwsProviderConfig {
Region = "us-east-1"
});
new Instance(this, "hello", new InstanceConfig {
Ami = "ami-2757f631",
InstanceType = "t2.micro"
});
}
public static void Main(string[] args)
{
App app = new App();
new MyApp(app, "hello-terraform");
app.Synth();
Console.WriteLine("App synth complete");
}
}
}
If you would like to use C# to generate Terraform configuration, check out the Getting Started guide for C#.
» Support for Java
Java support in CDK for Terraform allows users to write CDK constructs to define infrastructure in Java.
package com.mycompany.app;
import software.constructs.Construct;
import com.hashicorp.cdktf.App;
import com.hashicorp.cdktf.TerraformStack;
import imports.aws.AwsProvider;
import imports.aws.Instance;
public class Main extends TerraformStack
{
public Main(final Construct scope, final String id) {
super(scope, id);
AwsProvider.Builder.create(this, "Aws")
.region("us-east-1")
.build();
Instance.Builder.create(this, "hello")
.ami("ami-2757f631")
.instanceType("t2.micro")
.build();
}
public static void main(String[] args) {
final App app = new App();
new Main(app, "hello-terraform");
app.synth();
}
}
If you would like to use Java to generate Terraform configuration, check out the Getting Started guide for Java.
» Enhanced Support for Terraform Cloud
CDK for Terraform 0.1 adds enhanced support for Terraform Cloud that goes beyond the Terraform Remote State management features released as part of the project announcement in July. The project now supports Terraform Runs and Remote Operations in Terraform Cloud. This is enabled by using the Terraform Cloud APIs and follows the workflow-enabled by the API-driven Runs in Terraform Cloud.
The example uses a TypeScript CDK for Terraform project to showcase the remote operations in Terraform Cloud.
Let’s take a look at a CDK for Terraform application written in TypeScript that uses the null
provider to create a null
resource.
import { Construct } from 'constructs';
import { App, TerraformStack } from 'cdktf';
import * as NullProvider from './.gen/providers/null';
export class HelloTerraform extends TerraformStack {
constructor(scope: Construct, id: string) {
super(scope, id);
new NullProvider.Resource(this, 'test', {})
}
}
const app = new App();
new HelloTerraform(app, 'hello-terraform');
app.synth();
Currently, the application uses a local file to store Terraform state and all operations such as cdktf diff
, cdktf deploy
, and cdktf destroy
run locally.
Let’s add the remote state backend to allow Terraform state storage and run operations remotely in Terraform Cloud. Note that the Terraform Cloud workspace will need to be created and configured by the user using the console, Terraform CLI, or the Terraform Cloud APIs.
import { Construct } from 'constructs';
import { App, TerraformStack, RemoteBackend } from 'cdktf';
import * as NullProvider from './.gen/providers/null';
export class HelloTerraform extends TerraformStack {
constructor(scope: Construct, id: string) {
super(scope, id);
new NullProvider.Resource(this, 'test', {})
}
}
const app = new App();
const stack = new HelloTerraform(app, 'hello-terraform');
new RemoteBackend(stack, {
hostname: 'app.terraform.io',
organization: 'mishra-tf-cloud-demo',
workspaces: {
name: 'hello-terraform'
}
});
app.synth();
Run the cdktf diff
command locally. That will run a terraform plan
and generate a speculative plan using Terraform Cloud.
$ cdktf diff
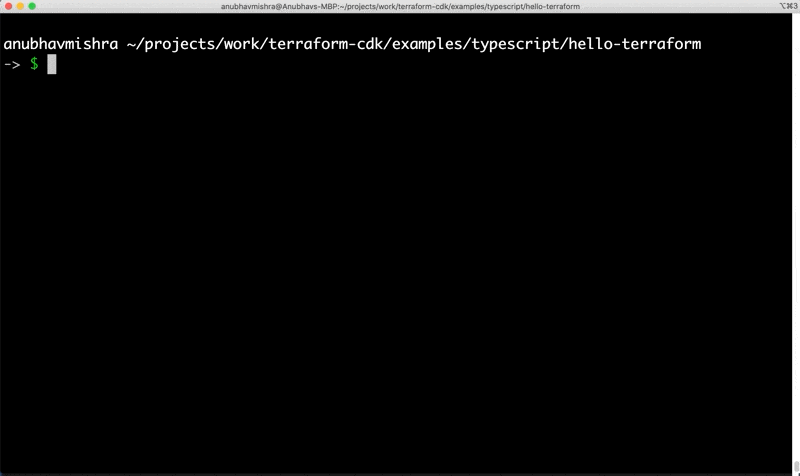
Next, deploy the application using cdktf deploy
. That will run a terraform apply
remotely using Terraform Cloud.
$ cdktf deploy
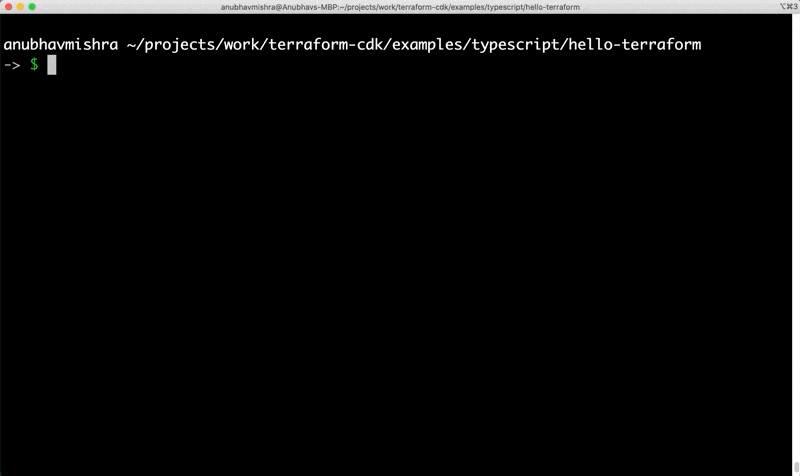
Using the remote state backend, you can run all Terraform operations that are run by CDK for Terraform remotely in Terraform Cloud.
» Sebastian Korfmann Joins HashiCorp
Today we are pleased to announce that Sebastian Korfmann, maintainer of CDK for Terraform, has joined HashiCorp as an employee. Sebastian will continue to focus on building CDK for Terraform and collaborating with the community on various features, reviewing pull requests and interacting in the HashiCorp Discuss forum.
Having Sebastian work on the project full-time will give us an opportunity to keep moving towards our goal of releasing beta and stable versions of the project.
Interested in working on CDK for Terraform, we are hiring!
» Available Now
The 0.1 release is available for download today! Try it yourself with our step-by-step examples using a Docker quick start tutorial, AWS, and other examples in Python, TypeScript, Java, and C#.
Since CDK for Terraform is still in alpha, we would like to ask the community for feedback. If you would like to see a feature for the CDK for Terraform, please review existing GitHub issues and upvote. If a feature does not exist in a GitHub issue, feel free to open a new issue. In addition to opening issues, you can contribute to the project by opening a pull request. Also, use the HashiCorp Discuss forum to ask questions and share your feedback.
Sign up for the latest HashiCorp news
More blog posts like this one
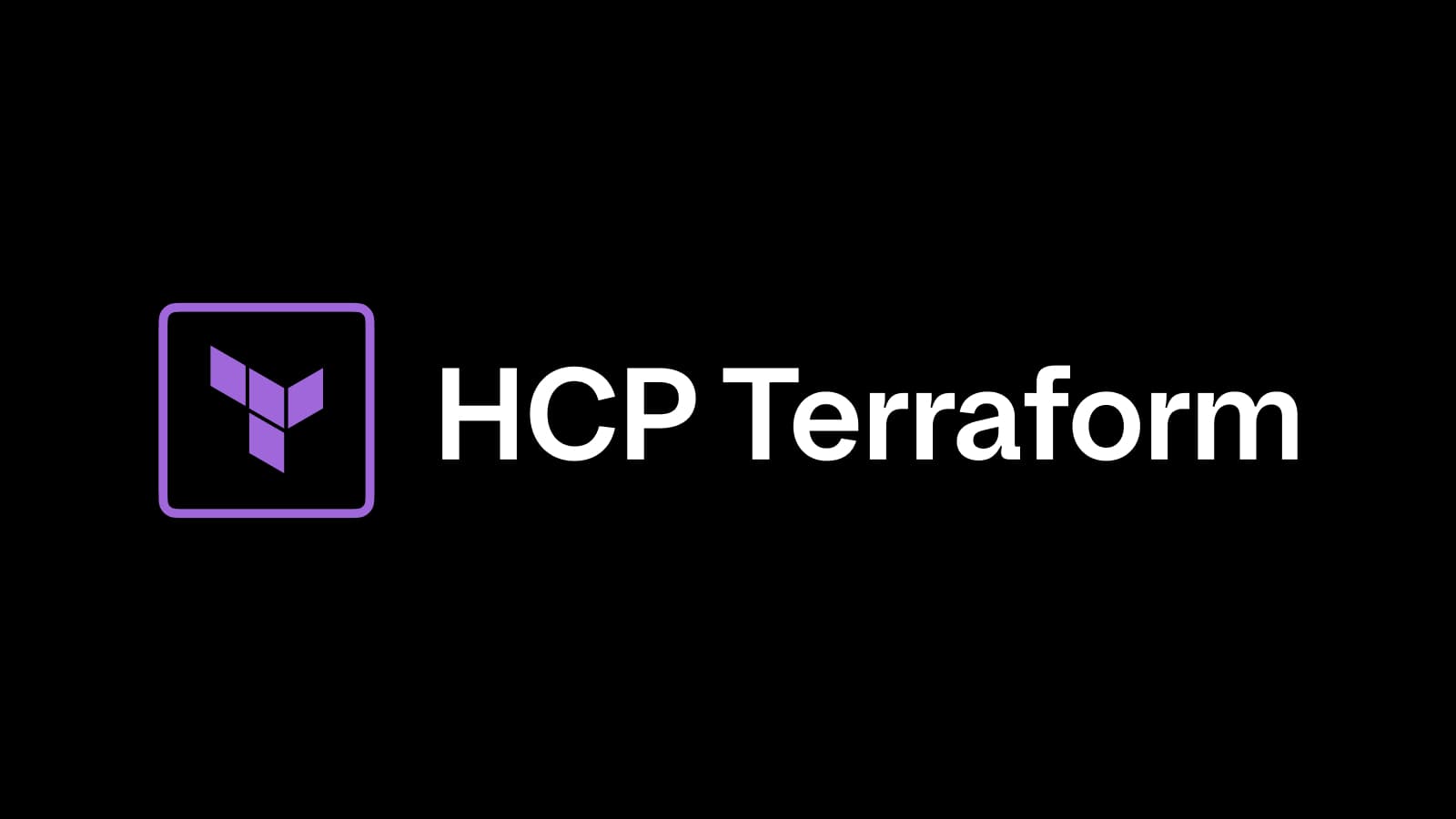
Terraform now supports multiple team tokens
Teams in HCP Terraform can now generate multiple API tokens per team, making multi-pipeline management easier and more secure.
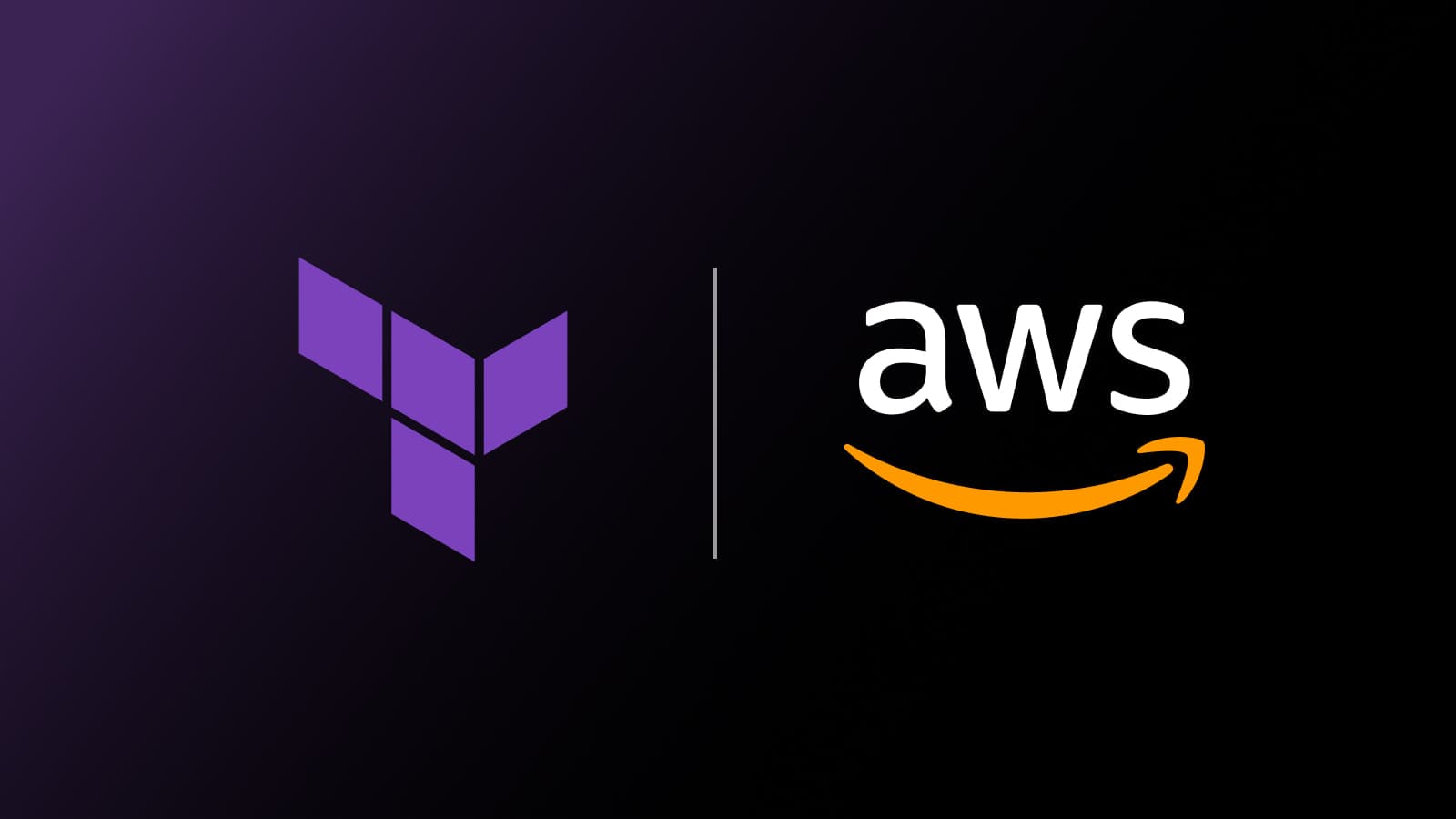
AWS and AWSCC Terraform providers: Better together
Manage your cloud infrastructure with the AWS and AWSCC Terraform providers and view strategies on how to move state between providers.
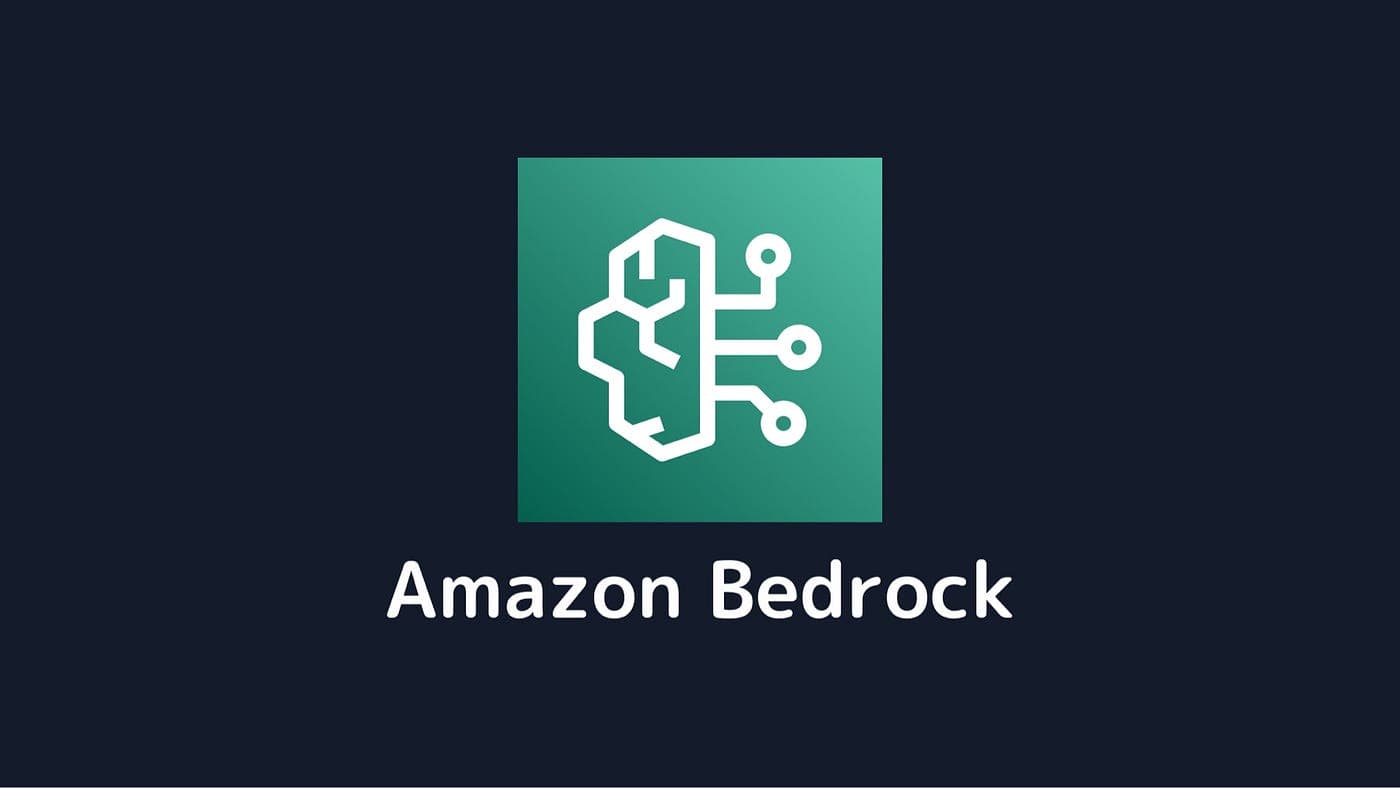
Protect data privacy in Amazon Bedrock with Vault
This demo shows how Vault transit secrets engine protects data used for RAG in an Amazon Bedrock Knowledge Base created by Terraform.